- Install Toolbox
- Install SQLite in GAC
- Install SQLite EF provider in project
- Run EDM Wizard
Once per Visual Studio edition (daily build at Install latest Toolboxhttps://github.com/ErikEJ/SqlCeToolbox/wiki/Release-notes )
Once per machine. Download sqlite-netFx46-setup-bundle-x86-2015-1.0.108.0.exe (from Install SQLite in GAChttps://system.data.sqlite.org/index.html/doc/trunk/www/downloads.wiki)
Select "Full Installation"
Select: Install the assemblies into the global assembly cache - Install VS designer components
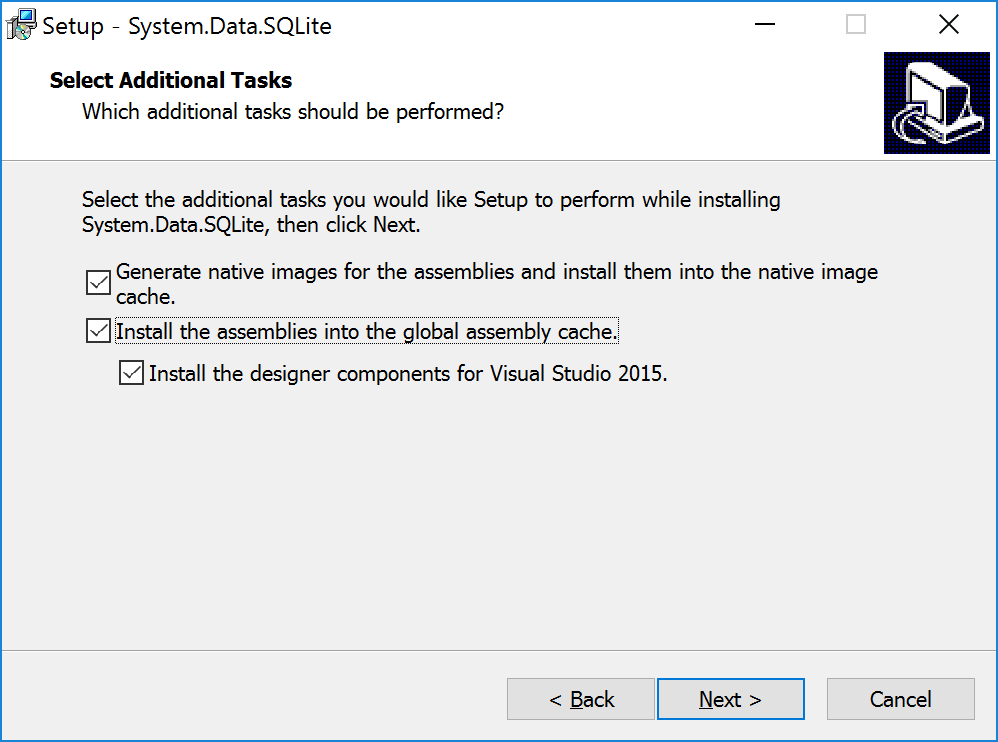
Restart Visual Studio
Verify that the EF6 provider is installed in GAC from the Toolbox "About" dialog:

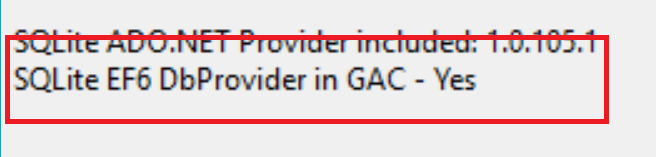
If the EF6 provider is not in GAC, this may be due to an invalid entry in machine.config, located in the C:\WINDOWS\Microsoft.NET\Framework\v4.0.30319\Config folder. The only SQLite related entry should look like this, with this exact version number:
<system.data>
<DbProviderFactories>
<add name="SQLite Data Provider"
invariant="System.Data.SQLite.EF6"
description=".NET Framework Data Provider for SQLite"
type="System.Data.SQLite.EF6.SQLiteProviderFactory,
System.Data.SQLite.EF6,
Version=1.0.108.0,
Culture=neutral,
PublicKeyToken=db937bc2d44ff139"
/>
</DbProviderFactories>
</system.data>
Install using Package Manager Console or NuGet Manager in each project. Install System.Data.Sqlite NuGet package
PM> Install-Package System.Data.SQLite
Make sure to install the same version as the tools package above.Build project!
Packages.config should look like this after install:
<packages>
<package id="EntityFramework" version="6.2.0" targetFramework="net461" />
<package id="System.Data.SQLite" version="1.0.108.0" targetFramework="net461" />
<package id="System.Data.SQLite.Core" version="1.0.108.0" targetFramework="net461" />
<package id="System.Data.SQLite.EF6" version="1.0.108.0" targetFramework="net461" />
<package id="System.Data.SQLite.Linq" version="1.0.108.0" targetFramework="net461" />
</packages>
<configuration>
<configSections>
<section name="entityFramework" type="System.Data.Entity.Internal.ConfigFile.EntityFrameworkSection, EntityFramework, Version=6.0.0.0, Culture=neutral, PublicKeyToken=b77a5c561934e089" requirePermission="false" />
</configSections>
<entityFramework>
<providers>
<provider invariantName="System.Data.SQLite.EF6" type="System.Data.SQLite.EF6.SQLiteProviderServices, System.Data.SQLite.EF6" />
</providers>
</entityFramework>
<system.data>
<DbProviderFactories>
<remove invariant="System.Data.SQLite.EF6" />
<add name="SQLite Data Provider (Entity Framework 6)" invariant="System.Data.SQLite.EF6" description=".NET Framework Data Provider for SQLite (Entity Framework 6)" type="System.Data.SQLite.EF6.SQLiteProviderFactory, System.Data.SQLite.EF6" />
<remove invariant="System.Data.SQLite" />
</DbProviderFactories>
</system.data>
</configuration>
Add, New Item, Data, ADO.NET Entity Data Model. Choose "EF Designer from Database" or "Code First from Database" Run Entity Data Model Wizard
Use "SQLite Provider (Simple for EF6 by ErikEJ)" when creating a connection to your SQLite database file.

No comments:
Post a Comment